Playing games in Roblox can be a lot of fun! If you’ve ever wondered how the creators of some of your favorite Roblox games have been so successful, then it may be time to go behind the scenes and learn to code your own games, too. Once you have installed Roblox Studio, you can play around with designing your own games and learning how to write scripts. Here is how to code for beginners in Roblox, with a short project included.
To build your Roblox coding skills with live expert instruction, and a curriculum designed by professionals from Google, Stanford, and MIT, join our free Roblox class - there's no risk in trying!
Learn how to code for beginners for Roblox
When you open a new game in Roblox Studio, there are unlimited options for what you can create! In this tutorial, we will show you how to add a fading platform to your game, one of the favorite challenges in any Roblox Obby.
1. Place a Part
Place a Part into your game where you’d like it to appear, and rename it FadingPart. Be sure it is big enough for a player to step on and anchor it on the Home Edit menu.
Add a script to FadingPart and call it FadesOnTouch. Be sure before you start entering in the code in the next step that you delete the default script when you open it.
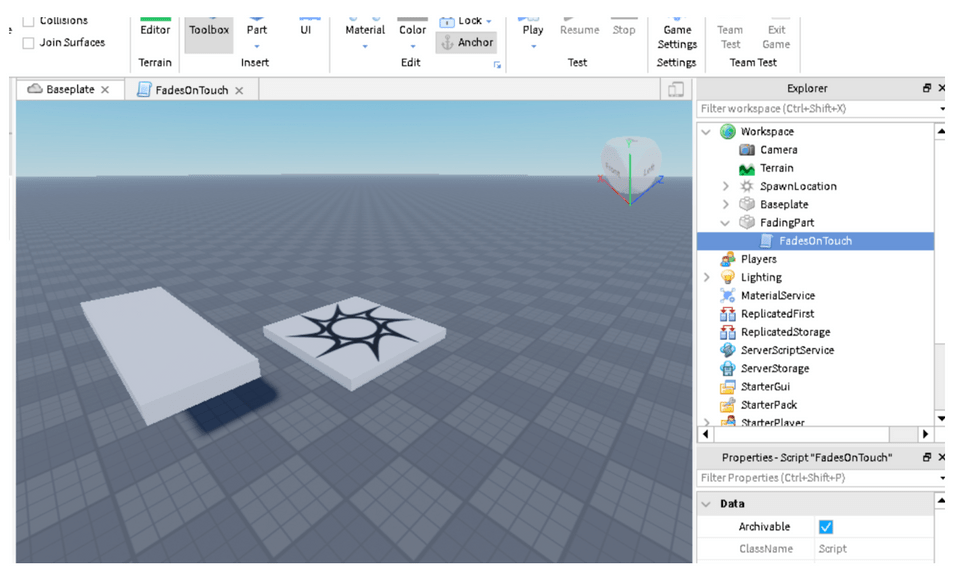
2. Create a variable
Create a variable for FadingPart (called platform) and add the following empty function and Touched command to the script:
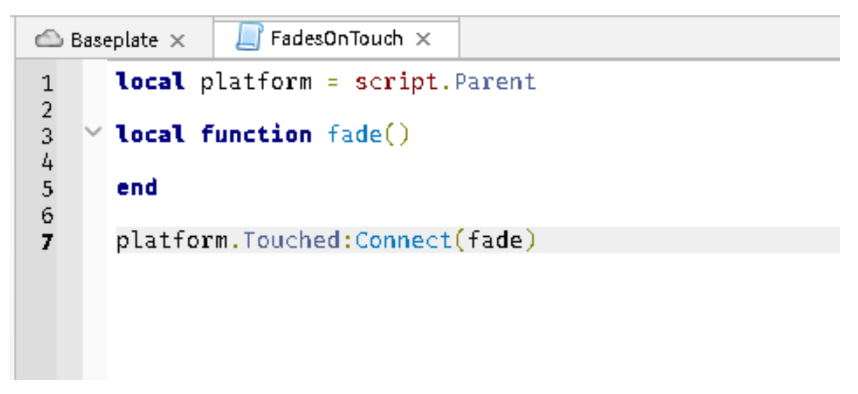
Be sure to note in the code above where there are parentheses and periods, they are important!
3. Place a For Loop
Next, we can place a For Loop inside the function that will cause FadedPart to fade away once it is touched. This line of code tells Roblox to start at a value of 1, end at 10, and step by increments of 1 to create the illusion of fading. Between each iteration of the loop, Roblox will wait for 0.1 second then loop again until it reaches the end value.
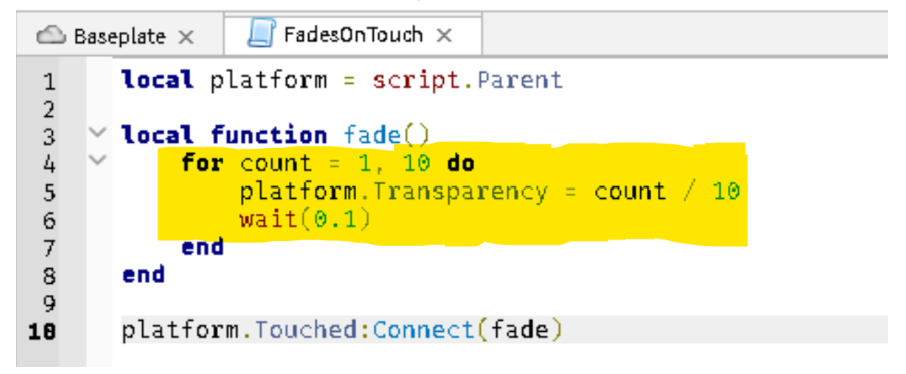
4. Make the player fall
Finally, we need the player to fall through the space where the platform was, which is a collision command. And of course, we will need the FadedPart to return (in this case, after 3 seconds) so the player can try it again and again:
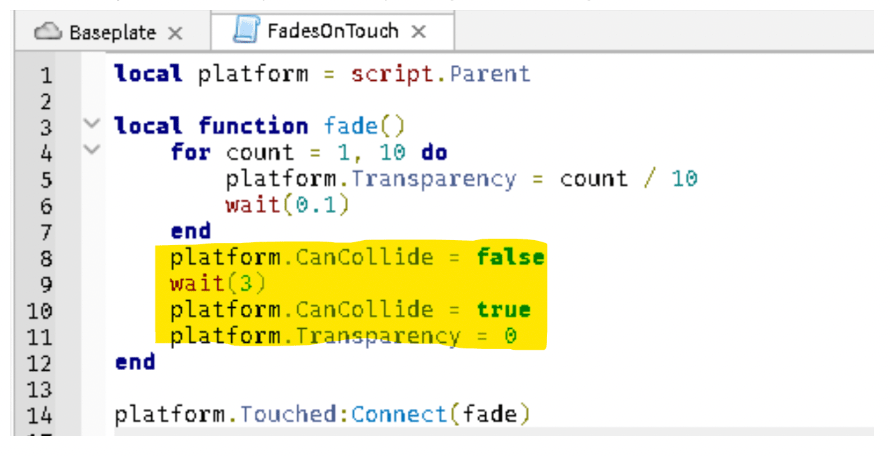
5. Try running it
Your finished FadingPart should run like this:
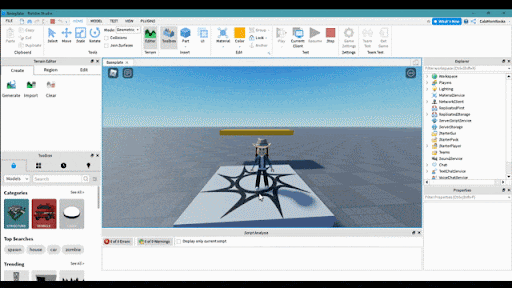
Roblox coding practice
Now that you know some Roblox coding basics, here are a few fun ways to get some Roblox coding practice! Try our helpful AI coach experience:
- Roblox Developer Hub: Roblox has a thorough tutorial section on their developer website that makes learning accessible to most ages.
- Online tutorials: Follow online Roblox tutorials such as this one on how to make a Roblox game.
- YouTube Videos: There are many Roblox Creators on YouTube that provide video tutorials on how to learn and use scripts in Roblox Studio.
Roblox coding course free
Taking an online course in coding can help students learn proper scripting skills and habits, with the benefit of a live expert who can answer questions along the way. Create & Learn provides a Beginner Roblox Game Coding class and Roblox camps, and each is taught by a professional, experienced instructor. Class sizes are very small, which allows for personal time with each student as they create their games. The best part is that you can start for free!
Now you know how to code for beginners in Roblox
Using this short script as a starting point, the possibilities are endless for creating your own fun games in Roblox Studio! When learning to write code, be sure to pay attention to the punctuation in the script, and also which letters are lower- and uppercase - it all matters in order for Roblox to run correctly. We encourage you to next read our Roblox Tutorial: How to Make a Game, so once you have your coding skills sharp, you can learn how to upload your game to Roblox for others to experience!
Written by Kari Tonkin, a Create & Learn instructor. Kari has been teaching a wide variety of courses and ages for more than twenty-six years. Some of her favorite subjects to teach include computer science, graphic design, mathematics, and coding languages. She received a Master’s Degree in Curriculum Design with Technology Integration from Black Hills State University in 2016 and has used this knowledge to stay current on new technology trends in education. When she is not working, Kari enjoys playing video games with her family, including Roblox and Minecraft, hiking and camping, and traveling around the United States.