Today, I will be sharing everything you need to know about variables in coding. Find out what variables are, how you can make them in a few different programming languages, and how they can be used effectively. Plus, I'll give you the opportunity to try a few fun variables challenges.
Discover How To Use Variables In Coding
What is data? How does the computer look at different types of data?
When we think about coding, something that may come to mind is messing around with a bunch of zeros and ones; this information that our programs store and interact with is called data! Data can come in a bunch of different forms, so here are a few simple ones:
- Integers are whole numbers, both negative and positive! Some examples: 1, 0, -325
- Characters are individual letters, symbols, and sometimes numbers that can be used to make up words or phrases. Some examples: “a”, “&”, “(“
- Strings are “strings” (or ordered series) of letters, symbols, and numbers that can make up words, sentences, phrases, and other useful material for computers and programs. Some examples: “Hello, World!” “Wow, I sure love coding!”, “This blog post has been brought to you by Create & Learn”
- Booleans are statements that the computer can check if they are true or false! These are very useful if you want your program to do certain things when certain conditions are met. Some examples:
- 50 > 40; the computer can check to see that 50 is, in fact, greater than 40, so it’s true!
- 700 < 10; the computer can check to see that 700 is not less than 10, so it’s false!
How can we store data for later? Variables!
This brings us to variables! Let’s say that we are creating a game where one of the player’s objectives is to get a high score. We will need something to store the number of points the player has that we can add to as the player plays the game, as well as a way to reset it back to zero once the player starts again.
What Are Variables In Programming?
Think of variables as a box that we can store things in, such as numbers, strings, characters, and a whole lot more! Variables can be created to store a particular value that we can add to overtime, but we can also completely replace it with something else if need be. Variables are also useful because they can act as a name for the information that it is storing, and we can get access to that data whenever we use its name! We’ll see some examples of its use below.
Examples Of Variables In Coding
Let's explore how to use variables in both beginner-friendly Scratch and Python coding.
Example of variables in Scratch coding
We’ll go back to our game example and add a little bit to it. Let’s make a game in Scratch where we have to catch Scratch Cat, and we get a point every time we click him! Let’s start by giving him some basic motion:
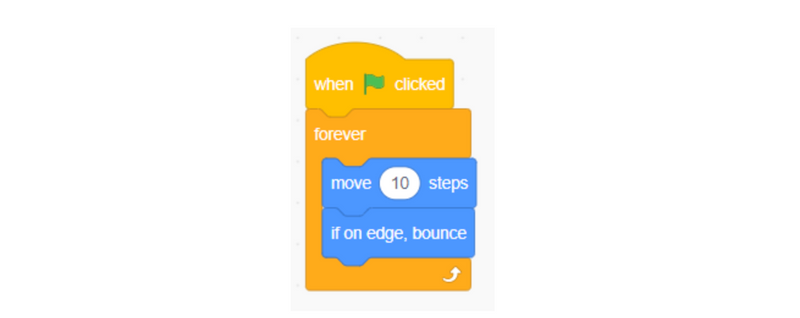
This will have Scratch Cat pace back and forth across the screen by looping through the instructions of moving ten steps, and bouncing if it is on the edge. Now, let’s make a variable to keep track of the score! We can do this by clicking the red circle that says “Variables” on the left side of the screen:
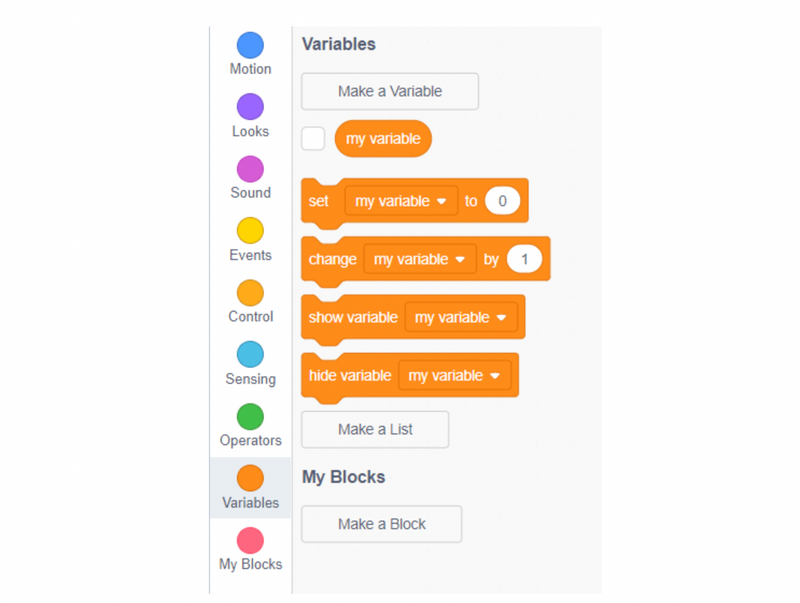
From here, we’ll want to click “Make a Variable” at the top. We can name variables anything we want, but it’s best to name it something specific so you can recognize what its purpose is! We’ll call this variable “Score”:
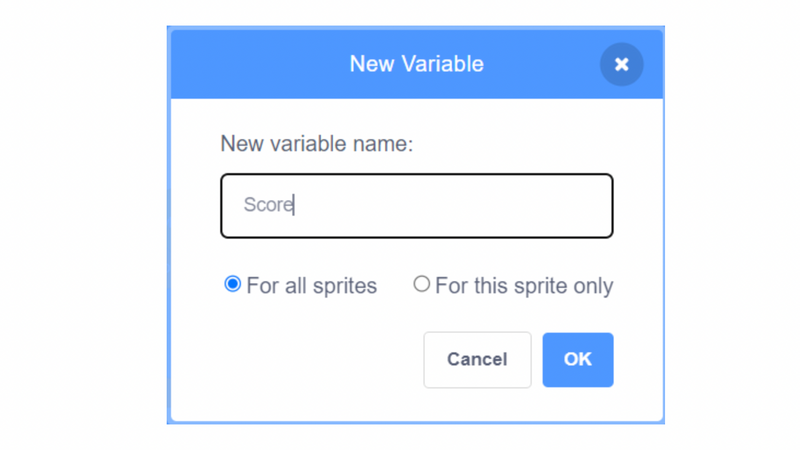
From here, there are two important blocks to take note of: the “Set Score to” block and the “Change Score by” block.
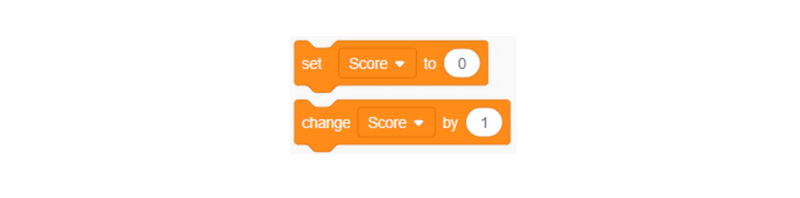
The “Set Score to” block is used to give our variable a value to store. Keep in mind that this will replace whatever the variable had before you used that block! The “Change Score to” block can be used to add or subtract from our score. If we put in a positive number where it says “1”, it will add that number to Score, and when we put a negative number there, it will subtract it.
Now, given that we want our score to always start at 0, we will edit our previous code so that “Score” resets to 0 at the beginning of the program:
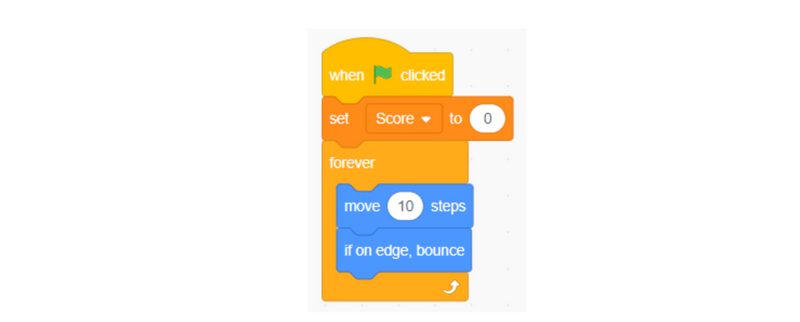
Great! The next step will be telling our sprite to add one to “Score” whenever it is clicked:

We have our game! Click the sprite to watch the number of points go up. Now, let’s add one final feature to our game: a timer! We will have the player get as many points as possible over the course of 60 seconds, have the sprite say their score, and then end the game. We can do that by editing our code like so:
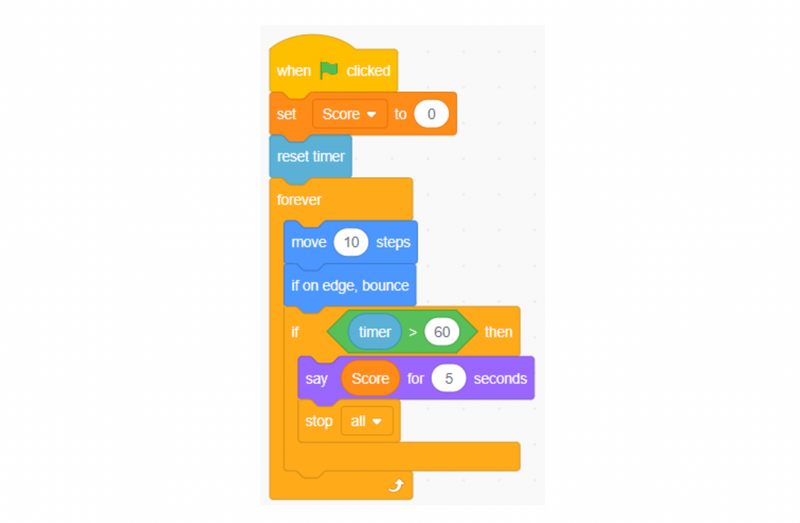
At the beginning of the program, we make sure to reset our timer, having it start at 0 seconds and counting up! While the sprite is moving, we make sure to constantly check if the sprite has made it up to 60 seconds. Once it hits that point and beyond, the sprite will use the “Score” block to say whatever value is held within that variable for 5 seconds. After that, the program stops all code and the game ends. Here is the example on Scratch if you’d like a closer look at it!
To learn more about how to use Scratch coding with live expert guidance, enroll your child in our award-winning online beginner-friendly free Scratch coding classes, designed by professionals from Google, Stanford, and MIT.
Example of variables in Python programming
Let’s see some variables in another coding language! If we want to make variables in Python, all we have to do is write the name of the variable and set it equal to whatever value you want stored. Let’s say that we want to make two variables, one called x that stores the number 30, and one called y that stores the word “Computer”. We can write those instructions like this:

Great! We can make variables in Python by simply writing the name of the variable and setting it equal to whatever value you want to store. We can use the names of the variables to refer back to their stored values! Let’s add a little bit more to our code:
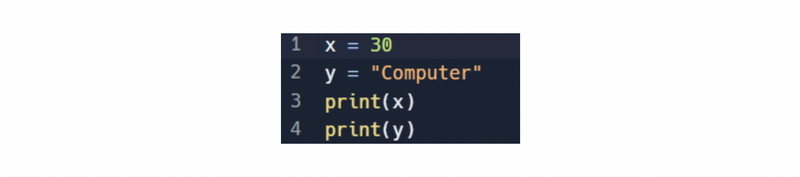
Notice that we’ve added “print()” to our code! In Python, print statements are ways that we can have the computer print out information that the user may want to see. Basically, it prints out whatever is in the parentheses onto the computer screen! When we run this code, we will see the following results:

The computer sees that we are printing out the variables x and y, so it checks what values are stored inside of them. Since 30 is stored in the variable x, it prints out 30 first, and since “Computer” is stored in the variable y, prints out “Computer” second.
Let’s make a slightly more complex program. This time, we’ll have the computer ask the user for a name, store that name in a variable, and print out a greeting with the name! The code will look like this:

We see a new function in our code: input()! In Python, input statements ask the user a question that they can respond to by typing in their answer. In this case, we write our question inside of the parentheses next to input. Since we are saying that the variable “name” is equal to that input statement, that means that whatever the user types in will be stored inside of “name”! From there, we are able to print out a greeting by adding some strings together to make a longer sentence using the information the user has provided. If I as the user typed in “Jessica” as my name, this would be what the computer prints on the screen:

How else could you use input()? What programs or applications can you think of that take input from the user?
To enjoy live expert guidance learning Python, enroll your teen in a fun free online Python class.
Try Some Variables In Coding Challenges For Kids
Now that we have gone over variables and how to use them, here are some ideas for programs you can make at home.
- Make a program that takes two numbers from the user and prints out their sum
- Make a program that makes mad-libs based on input from the user
- Make a program that asks the user different math questions and gives different output if they’re right or wrong
What other programs can you think of that you can make using variables?
Get Started With Variables In Coding
Hopefully you’ve learned a little bit about variables. If you’d like to learn more, explore how to use loops in Python. Get started making awesome games with free coding classes. Happy coding!
Written by Create & Learn instructor Jonah Zimmermann. Jonah has focused on creating and teaching computer science, coding, and STEM curricula for elementary and middle school students. In his free time, he enjoys video games and a good Dungeons and Dragons session with friends!