When we write programs, we are implementing algorithms to represent tasks and solve problems. Algorithms typically consist of sequencing, selection, and iteration. Sequencing is the order that commands are executed, and selection determines which tasks should be executed based on specific conditions. Iteration allows us to repeat commands and is also useful when working with lists and strings. One way that we can repeat commands in Python is using a for loop. Let's take a look at how a Python for loop works and the different ways we can use it in our programs.
Jump right into learning all about Python with an award-winning live online class led by an expert and designed by professionals from Google, Stanford, and MIT.
How to use Python for loops
A for loop is used to iterate over a sequence, whether that is a list, a string, or a range of values. We can use a for loop to execute a set of statements for each item in a list, letter in a string, or value in a range of values.
The general syntax for a for loop is:
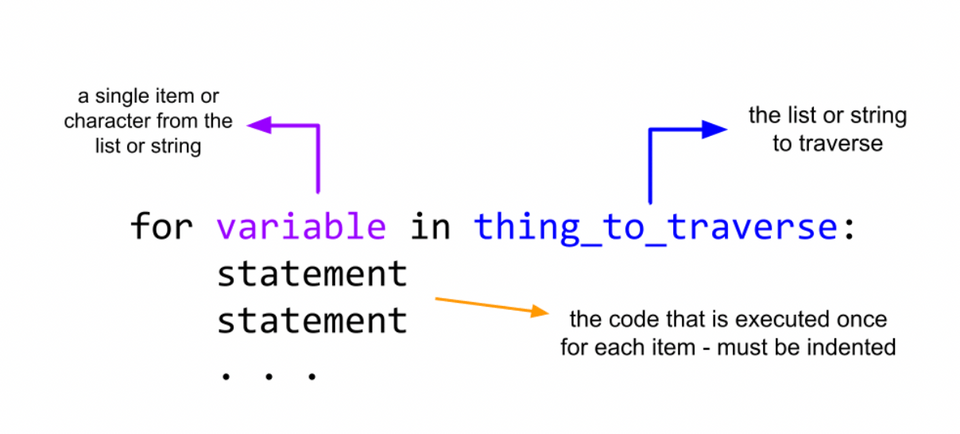
We start by writing the keyword for followed by a variable name that we want to use to refer to each item we are traversing. Then we write the keyword in followed by the name of the list or string we want to traverse. The colon ( : ) operator is used to specify an indented block of code.
The code we want to execute is indented under the for loop header. This code is executed once for each item in the list or string we are traversing.
Let's use a for loop to traverse a list of names. We can print each name as we go through the list.

With this for loop, we are printing each name in the names list. When we run our code, we see each name displayed.
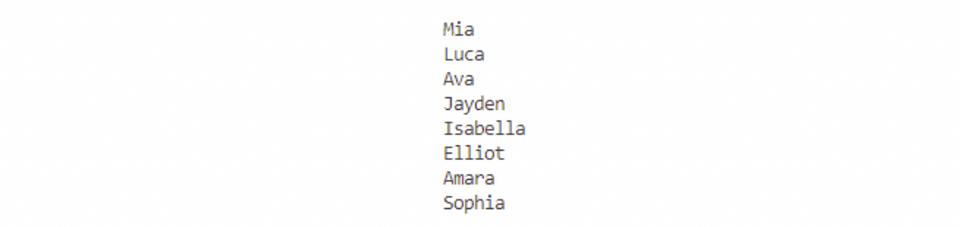
Strings are a sequence of characters. This means we can use a for loop to iterate through the letters in a string. For example, let's print each letter in the string "Coding is fun" using a for loop like this:

When we run this code, we see each character displayed on a separate line.
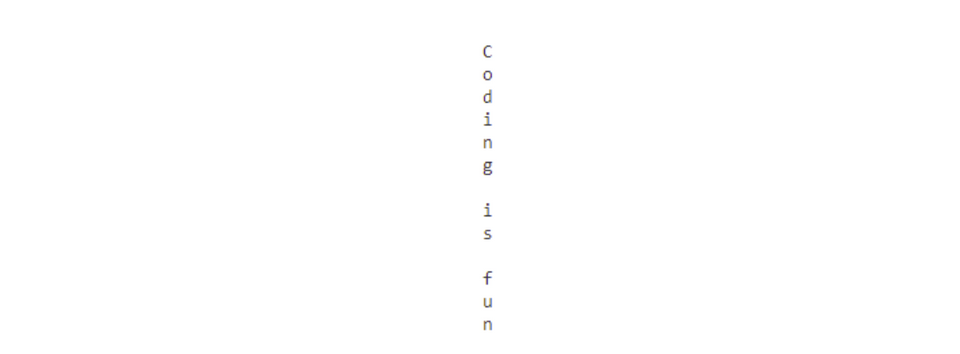
Sometimes we may want to stop a loop early before it has gone through all of the items in a list. We can use a break statement to stop the loop when a specific item is found.
For example, if we want to print each name until we get to "Ava", we can use a break statement inside of an if statement to check if the name is "Ava" and break the loop if this is true. We stop searching the rest of the list when we find "Ava".
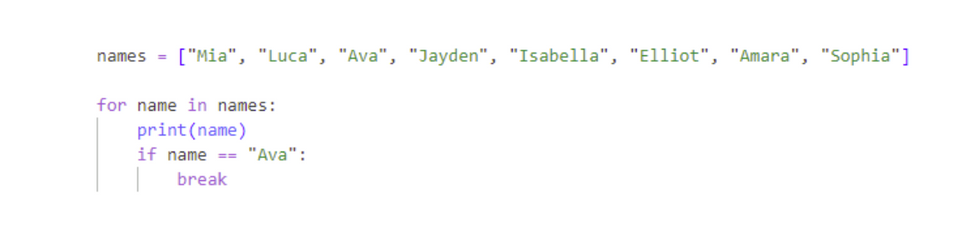
When we run this code, we see that "Mia", "Luca", and "Ava" are printed. The rest of the items in the names list are not printed.

What if we switch the order of these statements?

If we print after the if statement, then only "Mia" and "Luca" are printed. The break statement exits the loop before reaching the print function.

Python also has a continue statement that we can use to stop the current iteration of the loop and continue with the next. For example, if we find a specific name in the list, we can continue to the next name in the list instead of printing it.
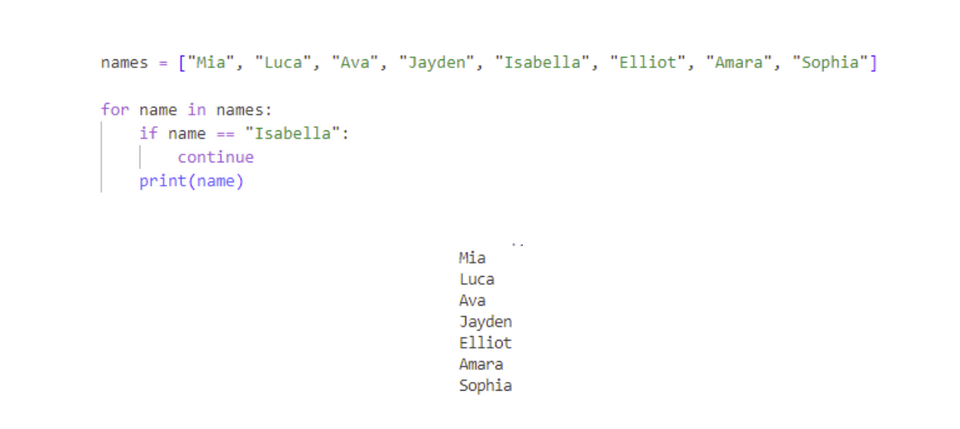
"Isabella" is not printed after "Jayden" because the continue statement stops the current iteration of the loop, skipping the print function.
A for loop can be used to repeat a block of code a specified number of times using the range() function. The range() function returns a sequence of numbers starting at 0 by default. It increases the value by 1 by default and stops at a specified number.
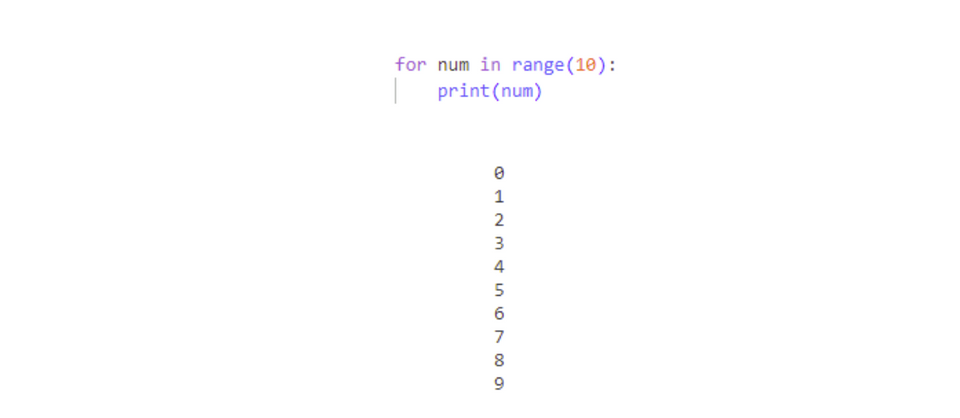
Notice that the range() function repeats up to but not including the specified number.
We can specify the value we want the range() function to start at by adding a parameter. The first parameter specifies the value to start at, and the second parameter specifies the value to stop at.
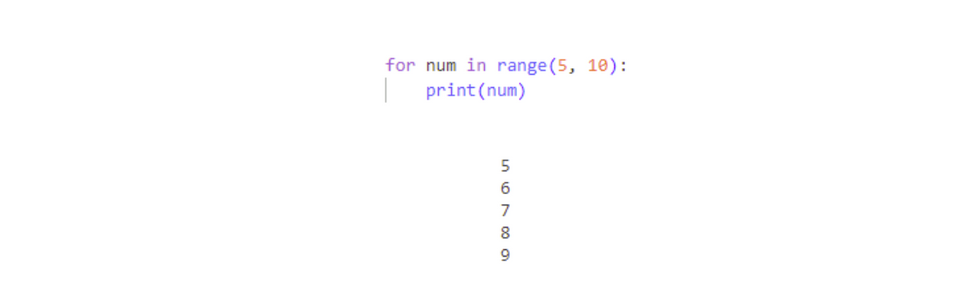
Finally, we can also change how much the value is increased by adding a third parameter to the range() function. By default, it increases by 1, but we can change it to increase by a different amount. The first parameter specifies the value to start at, the second parameter specifies the value to stop at, and the third parameter specifies how much to increment by.
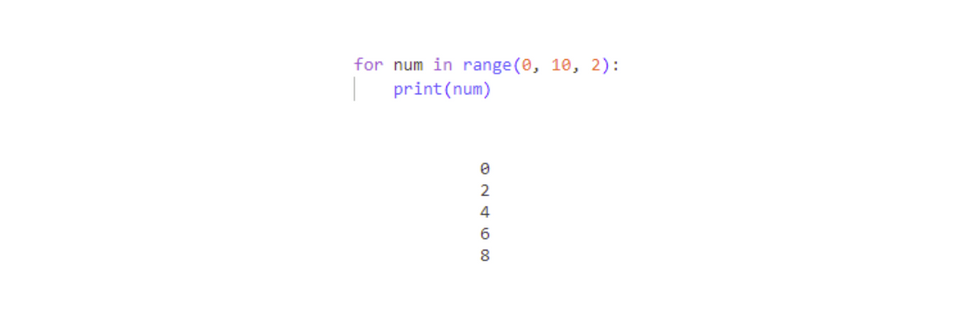
When we have multiple lists that we want to traverse at the same time, we can use nested for loops. A nested loop is a loop inside of another loop. The inner loop will complete all of its iterations before moving on to the next iteration of the outer loop.
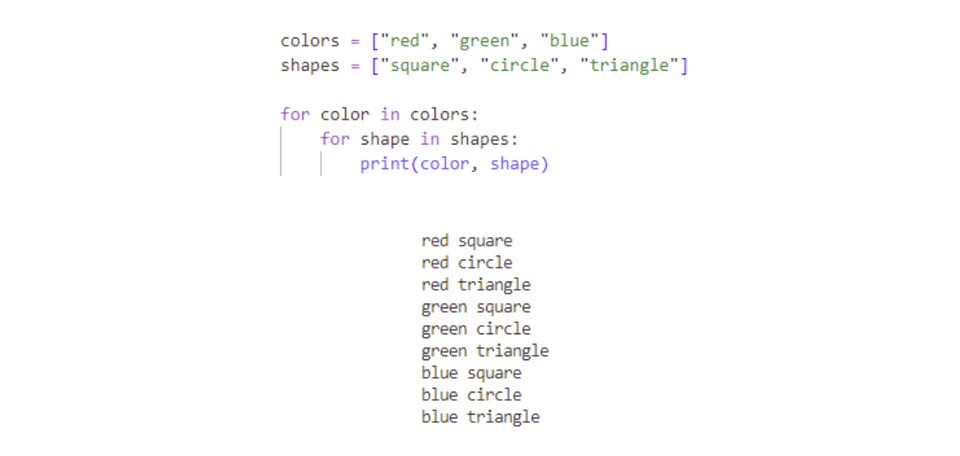
Use Python for loops
A for loop is a powerful tool for repeating a block of code and working with lists and strings. Loops make up a fundamental building block for writing algorithms and simplifying our code. You can learn more about the different ways we can use for loops in our award-winning online Python for AI course and Python camps, designed by professionals from Google, Stanford, and MIT! Join our free Python class to get started with live guidance from an expert.
Written by Jamila Cocchiola who has always been fascinated with technology and its impact on the world. The technologies that emerged while she was in high school showed her all the ways software could be used to connect people, so she learned how to code so she could make her own! She went on to make a career out of developing software and apps before deciding to become a teacher to help students see the importance, benefits, and fun of computer science.