Today, we’re going to explore a critically important programming concept - loops. Loops in coding are used in every major language, and allow us to write efficient, repeating chunks of code. In this article, we’ll look at the main types of loops, learn some general tips for writing loops, and go through some examples.
What Are Loops In Coding?
When you write code, your program executes from top to bottom. That means that each line only runs after the previous line has run. But sometimes, we want to write code that repeats. For instance, suppose you’re making a hide-and-seek robot that needs to count to ten before it starts to look for a player. In Python, you might write:
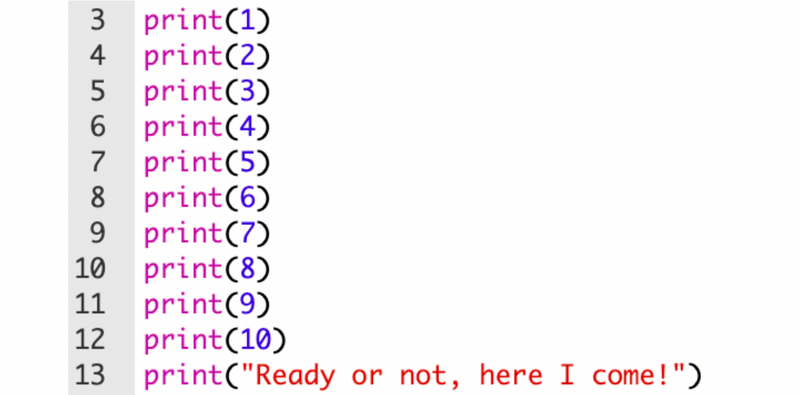
This code will print out the numbers 1-10. However it’s extremely repetitive, as the first ten lines are identical calls, except for the number being printed.
Loops allow us to write this type of code much more efficiently. Rather than write the same lines of code over and over, we can put this code in a loop and end up with a much shorter, cleaner program.
In most languages, there are two main types of loops, for and while. For loops repeat a chunk of code for a specified number of times. While loops repeat a chunk of code while some condition is true, stopping when the condition is no longer true. Some coding languages, like Scratch, also have until loops, which are the opposite of while loops; until loops run until a condition is true.
Examples Of Loops In Coding
Shown below are some examples of loops in both Python and Scratch.
Scratch example of loops
Two example loops are shown in the image below:
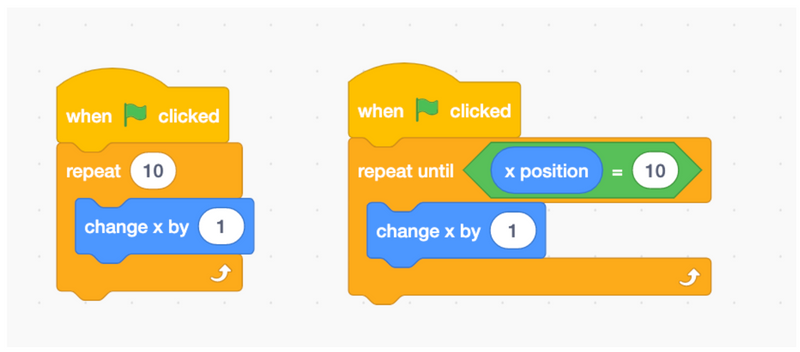
The code blocks on the left represent a for loop. The variable, x, increases by one ten times. The example on the right is an until loop. The right set of blocks will execute until x is ten. If x starts from zero, both of these code blocks will produce the same result.
Enroll your child in our live online, expert-led free Scratch classes for guidance on loops and much more to make cool games and animations:
Python loop example
For and while loops can also be written in Python. Returning to our hide-and-seek example, a three-line for loop can be written to replace all 11 lines of code:
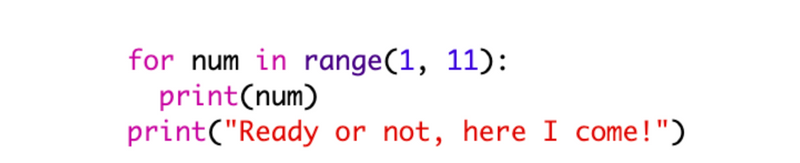
We can write an equivalent while loop that produces the same results.
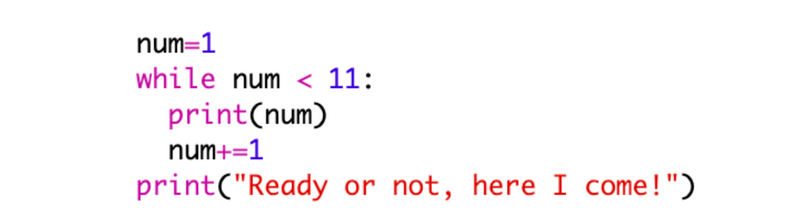
Both examples are many fewer lines, and much easier to work with than our previous example. Learn how to write Python for loops here.
Enroll your teen in our live online free Python class designed by experts from Google, Stanford, and MIT to learn more about harnessing this real-world language:
How To Use Loops In Coding
When you want to write code that repeats, the first thing to think about is your choice of loop type. In many cases, like those shown above, either a for or a while loop can be written. However, often one type of loop is more suited to the problem. For loops are great when you know exactly how many times you want to repeat a piece of code. For loops are also helpful when you want to iterate through a list, repeating the same action on every item in the list.
While loops are better when you don’t know how many times you need the loop to run. Suppose you want to make a game that repeats while a user does various actions, and stops when the user wins. You don’t know how long the user will play, but you do know under what conditions you want to stop the game. A while loop is well-suited to this type of problem.
Here’s some things to think about when building loops:
- For loops need to have their range defined ahead of time. In Python, you can use range(x,y) to write a loop that runs from x until y-1 (y is not included).
- While loops need to be initialized. That means that before the loop runs, we need to set a variable that will allow us to enter the loop. In the Python code above, I set num=1 before starting the loop. The condition, num<11, is satisfied and the loop starts to run. If I set num=12, the loop would not run because 12 is not less than 11.
- While loops can accidentally run forever if the condition is always satisfied. When this happens we’ve created an infinite loop, and have to manually stop our program. For instance, in the Python code above, after we print num, I add 1 to num (num+=1). Eventually, num will be greater than 11 and the loop will end. But if I took the line num+=1 out, num would always be 1, and the loop would run forever.
Challenges: Practice Using Loops In Coding
Want some practice using loops in coding? Try the following challenges in your favorite language:
- Create a list of your friends’ names. Write a loop that greets each of your friends: “Hi Annie!”, “Hi Bob!”, “Hi Conrad!”...
- Write a loop that starts at 1, and adds the previous number to the last number. If your program is working, you’ll get 1, 1, 2, 3, 5, 8… This sequence is called the Fibonacci sequence.
- Write a loop that returns the first 20 square numbers.
Get Started With Loops In Coding
Today, we dipped our toe into the concept of loops. Loops are one of the trickier concepts for beginner coders, and require some practice before they come naturally. If you’d like to learn more about loops and other coding concepts, check out our award-winning free coding classes to get started. Up next, learn about conditionals in coding.
Written by Sarah Rappaport, who graduated from Northwestern University with undergraduate and graduate degrees in engineering and music. She's now working on a masters in data with Georgia Institute of Technology. She taught math and computer science with Teach for America for two years, and now works as a Systems Engineer.