When we’re writing code, we often need to check to see if the user has done something, or if some particular event has happened, and then respond to that event in a specific way. To accomplish this, we can use conditional statements. This is a vital part of your coding repertoire, so read on to learn all about conditionals in coding!
What Are Conditionals In Coding?
To put it simply, conditional statements are blocks of code which respond to certain states in our program. We can use conditional statements to check for nearly any state. For example, maybe we want the program to save a file when the user hits a hotkey. The condition here is the hotkey being pressed, and the action to follow is saving the file. Or perhaps we want a character to jump when the player presses the jump button. The condition is the jump button being pressed, and the action is the jump. We can also check other aspects of the program. For example, the condition to be met might be making sure the player’s health is greater than zero. If not, then it’s game over!
Almost every programming language has some form of conditional statement the coder can use to control the flow of their program. From C++ to Python to Scratch, they all have way to check and respond to conditions in your program.
How To Use Conditional Statements In Coding
To check to see if a certain condition has been met, most programming languages use if statements. In plain English, we’d say:
“If a certain condition is true, then our program will do this.”
We can also use additional conditional statements, such as else if and else, to further control the logic flow by adding additional conditions to be checked at the same time. In plain English:
“If condition A is true, then do this. If condition A wasn’t true, then if [else if] condition B is true, then do this instead. If none of those conditions have been met [else], do this.”
You can see a couple of examples of what this might look like below. Every coding language has a slightly different syntax, but they all accomplish the same goal of responding to various states in your program.
Here are some things to keep in mind when using conditional statements:
- Conditional statements must check for true or false conditions.
- Conditional statements only check that condition once. If you need to check a condition over and over again, you’ll need to use loops.
- There must be a clear action to take if the condition is met (for example, pressing the jump button has a single, clear outcome of jumping).
- There’s usually a way to check for more than one condition at a time if needed.
To learn more about how to use conditionals with live expert guidance, enroll your child in award-winning online beginner-friendly free Scratch coding classes, designed by professionals from Google, Stanford, and MIT.
Examples Of Conditionals In Coding
Let’s have a look at a couple of examples of conditional statements—one in Scratch, and one in Python. Although these languages are very different from each other, you’ll see that condition statements look very similar!
Scratch conditional example
Here’s an example of a simple conditional statement in Scratch. Here, we have the Scratch Cat ask the user what their favorite color is. If the user says their favorite color is blue, the Scratch Cat responds that it’s also their favorite! If the user says their favorite color is red, the Scratch Cat says it’s their best friend’s favorite. If the user says anything else, they respond that it’s a great color.
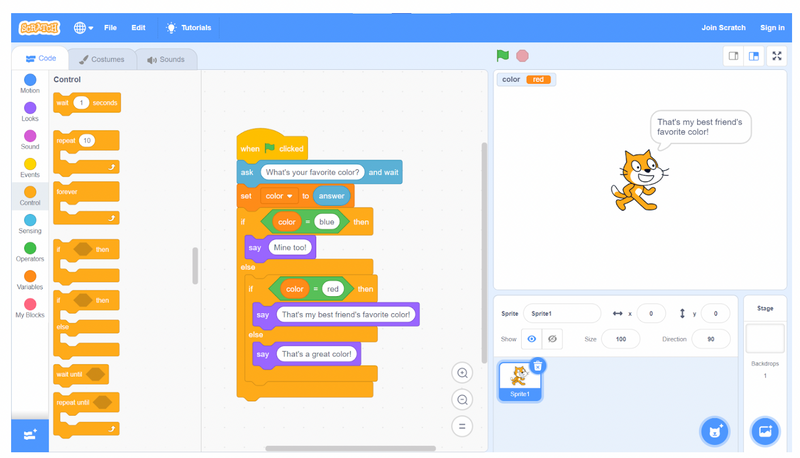
Scratch unfortunately doesn’t have any form of else if statement, so we must nest our additional conditional checks within the else statement of the first if-else block. It might sound a little confusing, but think of it this way: we check our first condition, but if that one wasn’t true, we move on to the else block. We then check the next condition. If that one isn’t true either, we move on to its else block, and so on. We can chain these together as much as we need to!
It’s worth noting that Scratch has some extra logic behind the scenes to ensure that the user’s response is easy to check with conditional logic. Even though we’re specifically checking to see if the user said “blue,” the user could add extra spaces before and after their answer, write their answer in all capital letters, or any number of other variations, and Scratch can still run the if statement without issue.
Python conditional example
Here’s an example of the same conditional checks in Python. We first ask the user for their favorite color. Then, using an if-elif-else block, we check to see how the user responded. As with the Scratch example, if the user said their favorite color was blue, we reply that it’s ours too! If they said it was red, we respond that our best friend shares that favorite. Otherwise, we let them know it’s a great color.
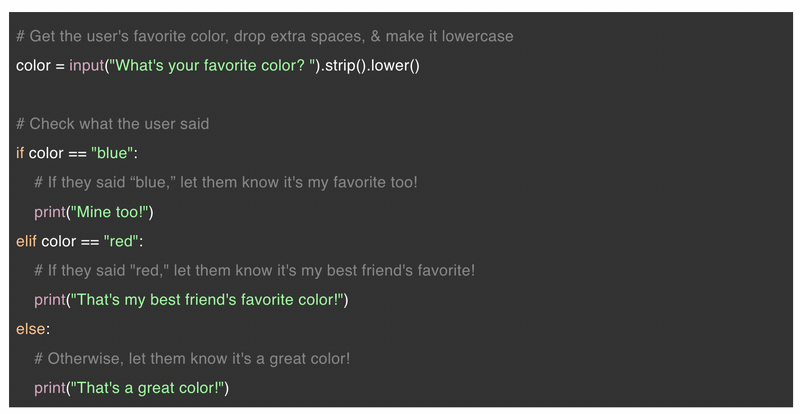
Unlike in Scratch, here we can check any number of conditions we’d like using the elif (short of else if) statement. We can have as many elifs as we want following the initial if, and Python will check each statement one by one until it finds a true statement. If it doesn’t find any true statements, it’ll run the code under the else statement.
In Python, we must be a little more specific with our conditional checks. Where Scratch was happy to check a user response of “blue,” “Blue,” or “BLUE” all as the same thing, in Python, those three iterations are considered to be completely distinct from one another. We solve this problem by using .strip() and .lower(). Adding these methods onto the end of our input statement gets rid of any extra spaces the user may have accidentally typed, and converts whatever they type into all lowercase letters. This way, we only have to check one version of the answer, rather than every possible capitalization the user could have typed!
To learn even more ways to control the program flow in Python, check out our blog post on Python loops! Your child might also enjoy learning Python programming in an award-winning live online free Python class.
Programming Conditionals
Now it’s your turn! If you prefer Scratch, try having the Scratch Cat respond to all sorts of different questions, or even have it respond to other events, such as key presses!
If you’re more of a Python person, try creating a few different questions to ask the user, and respond appropriately. You could create a whole conversation with conditional logic.
Get Started With Conditionals In Coding
Conditional statements are a foundational part of programming. A good program responds to the user’s input, and the best way to do that is with conditional logic. Need an idea of where to start practicing with conditionals? Check out our blog post on how to make a Tic Tac Toe game in Scratch.
Written by Create & Learn instructor Josh Abbott Salazar. Josh is a teacher, coder, audio engineer, and musician. After graduating with a Master's in Music from Belmont University in Nashville, TN, Josh turned his attention to the technology side of things, and has been working in various aspects of coding and engineering ever since. He runs a small music studio in Nashville called Tango Sound Studios, and develops video games in his spare time.