In programming, we often run into situations which call for code we’ve already written somewhere else. Sure, we could just copy and paste the code from earlier to accomplish the new task. But there’s a better way! Object oriented programming (OOP) allows us to create multiple copies of the same code with a single line, and best of all, we can make as many copies as we need. Almost everything in Python can be an object, so read on to learn more about how object oriented programming can level up your coding.
Learn all about Python in live online, small group classes, led by experts and designed by professionals from Google, Stanford, and MIT.
Discover the Advantages: Learn Object Oriented Programming
Object oriented programming is a powerful tool, and it’s easy to learn! It only takes a little practice to master this incredibly useful coding skill. Follow these simple steps to get started with OOP.
Note: If you need to see all of the code in context, the complete code can be seen at the bottom of this post.
1. Create a Class
To get started with object oriented programming, we’ll first need to create a template from which our objects are created. These templates are called classes.
To create a class, we use the class keyword, followed by the name of our class, then a colon. Indented inside our brand new class, we can name some variables to give our class some properties.

2. Create an Object
Once we’ve created a class, we can create as many objects from that class as we’d like. Each object we create is called an instance of the class, and is created outside of the class indentation structure. Notice the parentheses following the class name as we call it—these are important, so be sure not to forget them!

Once an instance of a class has been created, all of the information about that class can be accessed by calling the instance, followed by a period, followed by the property we need. We can use this to either get the information or change it! If we add the following to our code…
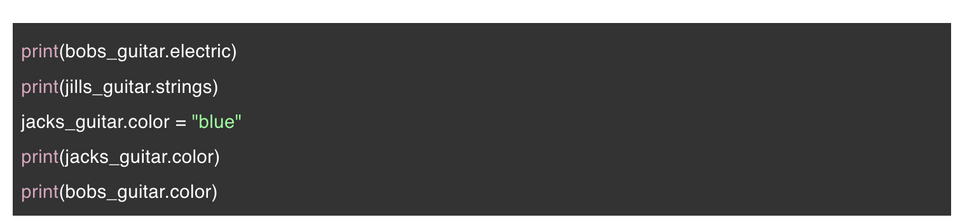
…and run it…

…we’ll see that we were not only able to print out the properties we asked for, but we were able to change the color of Jack’s guitar without affecting the color of the others!
3. Add a Method
Keeping track of properties is just the beginning of what classes can do. In addition to that, we can also use classes to keep track of functions related to the class. When functions exist inside of a class, we call them methods. To write a method, we use the def keyword as we usually do for functions, followed by the name of the method. Importantly, we must also include self as the first parameter (more on this later).
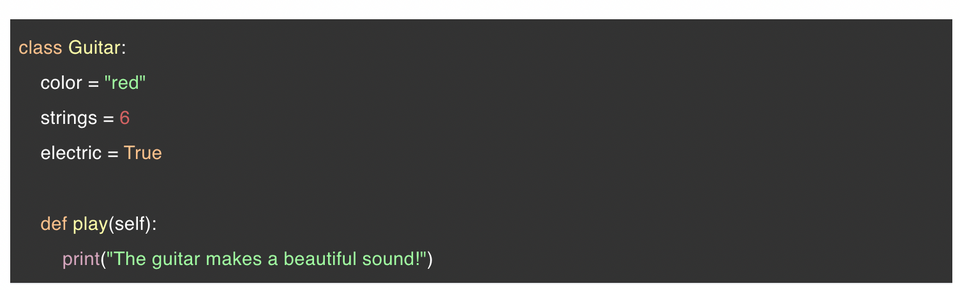
Once our class has a method, we can call that method the same way we called properties. So if we add this line to our code (after the creation of Bob’s guitar, of course!)…

…and we run it, we’ll see that our method called play happily ran for Bob’s guitar!

4. The init Method and the self Parameter
Within classes, we can also create special methods called magic methods, also known as dunder methods. Magic methods start and end with the double underscores, and they have special properties. The most common magic method is the __init__ method, which automatically runs the moment we create an instance of a class without needing to be called.
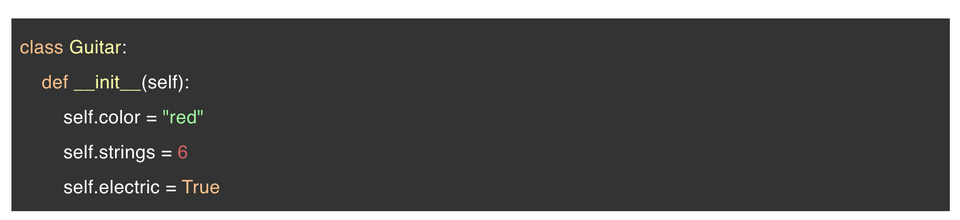
The __init__ method is where we typically define our properties in well-written code. Notice our properties now all have self. attached in front of the variable name. The self parameter is a reference to the current instance of the class. It solves the problem of scope within our class—that is, it allows us to access these variables inside of any method in our class by simply adding self. in front of the variable name. Let’s adjust our play method to take advantage of this:
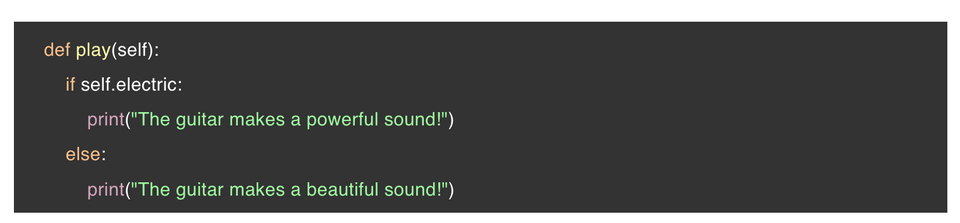
Because self.electric was defined inside of our __init__ method, and because it has self. tacked onto the front of it, it’s available for use throughout the class, including inside of our play method!
5. Passing Arguments Into the Class
What if we need to be able to set properties when we create a class, rather than being stuck with default values? To do that, we can ask for parameters in the __init__ method! After the self parameter, we can add as many parameters as we’d like. We must be careful, though! Those parameters will only be available within the __init__ method. Fortunately for us, that’s an easy problem to solve. We can expose the variable to the rest of the class by storing it in a self. variable.
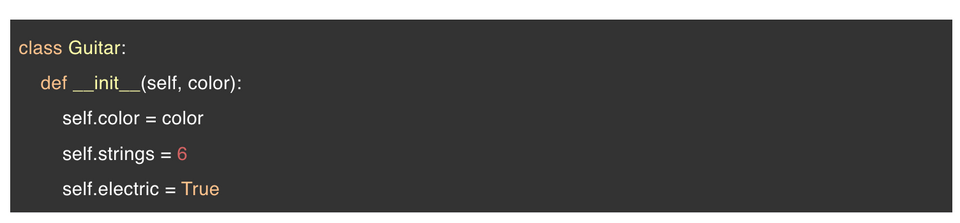
And later on in our script, outside of the class, we can pass through an argument called color whenever we create our class, like this:

Now, Bob’s guitar’s color will be red, Jill’s will be green, and Jack’s will be black! Notice in our class that we changed the self.color variable to be set to the value of color. This means whatever we passed through for the color argument when we created an instance of this class will be stored in self.color, and will therefore be accessible throughout the rest of the class. This is an important step when accepting parameters for our classes!
6. Inheritance
One of the most powerful things about classes is the concept of inheritance. Inheritance allows us to create a class based on an already existing class. The existing class, called the parent class or the base class, passes on all of the code within it to the new class, called the child class or the derived class. To create a child class, we simply name the parent class in parentheses right after the name of our new class:
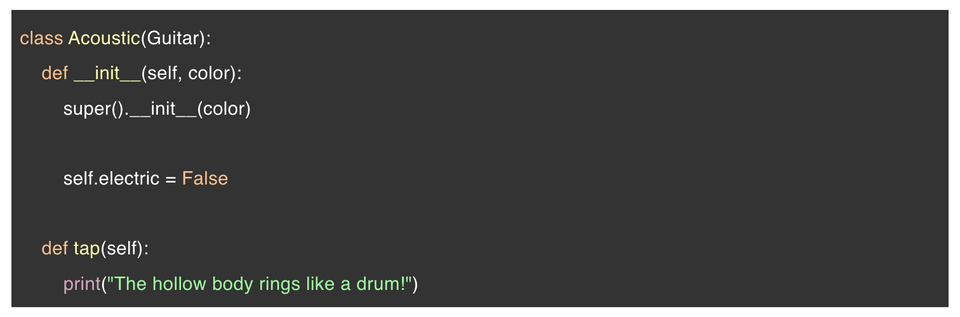
There’s a bit to unpack here. Notice the super().__init__() line? Whenever a child class has a method of the same name as one in the parent class, the child class’s method is given priority. That means if both the child and parent class have an __init__ method, only the child’s __init__ will run! To solve this problem, we can use the super() function to refer to the parent class, and explicitly ask to run the parent class’s __init__ as well. This is extremely common to see in child classes, so be sure not to forget that particular line of code! Notice also that we pass our color parameter on to the parent class in our super().__init__() line. Don’t forget that the parent class needs to know what color means, too!
Any child class can be said to contain all of the information of the parent class, as if that information had been copied and pasted into our new class. That means the play method from the parent Guitar class exists inside of our new Acoustic class, too! So if we add this to our code…

…and run it…

we can see that Python is just as happy to run the parent class’s play method as it is to run the child class’s tap method!
The Complete Code for our Object Oriented Programming Tutorial
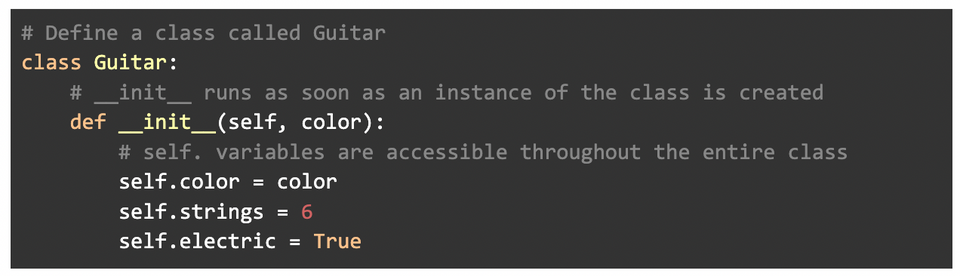
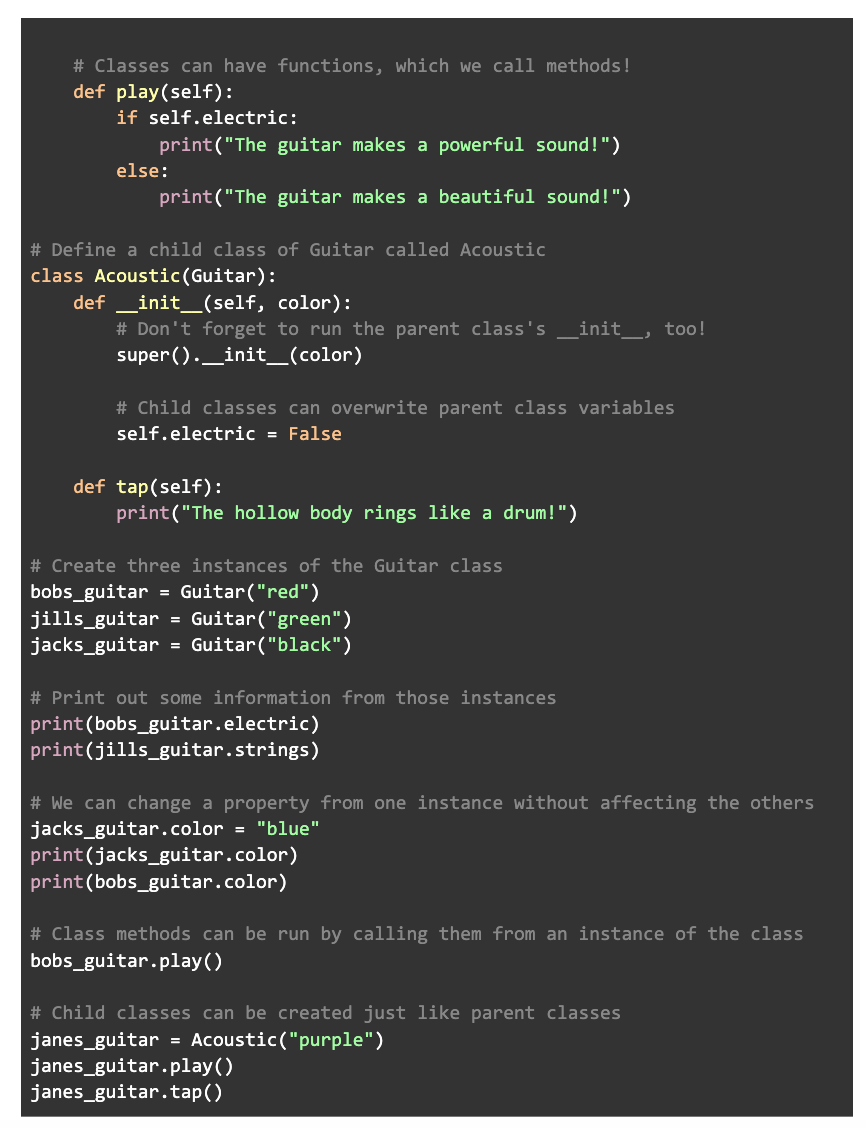
Learn Object Oriented Programming with Python
There’s even more to explore with Python and object oriented programming. Check out our blog posts on Python lists and Python for loops.
And don’t forget, you can also learn how to create all kinds of fun and interesting programs with Python by joining our live online, small-group, expert-designed Python classes! Begin with a free Python class, designed by professionals from Google, Stanford, and MIT - there's no risk in trying!
Written by Create & Learn instructor Josh Abbott Salazar. Josh is a teacher, coder, audio engineer, and musician. After graduating with a Master's in Music from Belmont University in Nashville, TN, Josh turned his attention to the technology side of things, and has been working in various aspects of coding and engineering ever since. He runs a small music studio in Nashville called Tango Sound Studios, and develops video games in his spare time.