Variables are fundamental components of a program to store and work with single values. Sometimes we have multiple values or lists of information that we need to work with. In Python, we can use a list to store multiple values. Using a list in our programs allows us to use one variable to refer to multiple values and easily search for information in the list and perform calculations. Let's take a look at how to use Python lists. To learn more about Python with live expert instruction, join our free Python class.
How to use Python lists
In Python, we create a list by placing the values we want to store inside square brackets [ ]. Each value is separated by a comma. Each value in a list is often referred to as an element.

A list can have as many items as you want, and it can store integers, decimal values, strings, or objects. Each element is stored at an index in the list. In Python, the indexes start at 0. So this list has five elements, and the elements are at indexes 0 to 4.
There are several ways that we can access the elements in a list.
We can use square brackets [ ] and the index where the element is located to access the value.
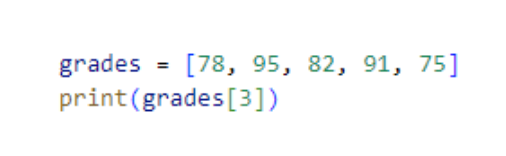
Output:

Lists are mutable, which means their elements can be changed. We can use the assignment operator = to change a value in the list.

Output:

Lists are objects, which means they also have functions we can use to work with lists. For example, we can use the append() function to add an item to the list. The append() function will add the given value to the end of the list.

Output:

We can also use the insert() function to insert an item at a specific index in the list. We first specify the index where we want to place the value then the value to insert at that location.

Output:

If we need to remove an item from the list, we can use the remove() function. The remove() function will remove the first occurrence of the given value from the list and shifts the rest of the list forward.

Output:

Now that we have data stored in a list, we can use loops to iterate through each item in the list. Let's print each value that is in the list using a for loop. We write the for loop to access each item in the list grades and print each item.

Learn more about for loops in Python.
Using loops and lists together, we can search for specific values in the list. For example, let's find and print any values that are greater than 90. Inside the for loop, we can use an if statement to check if the item is greater than 90. If this is true, then we will print the item. If this is false, we will do nothing.
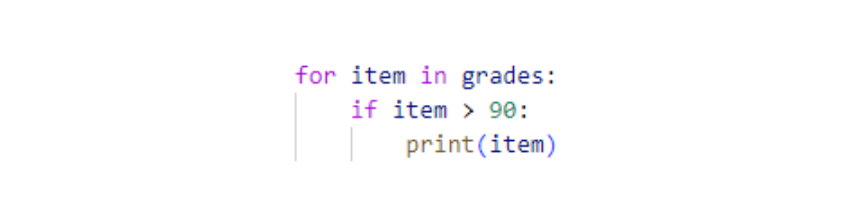
Output:

We can also perform calculations, such as finding the average of the values in the list. To do this, we start by creating a variable called sum to store the total of all the values in the list. We then iterate through the list and add each value to sum.
To find the average, we need to divide sum by the total number of values in the list. Python has a len() function that returns the number of items in a list. We can use this to divide sum by the length of the grades list and print the result.
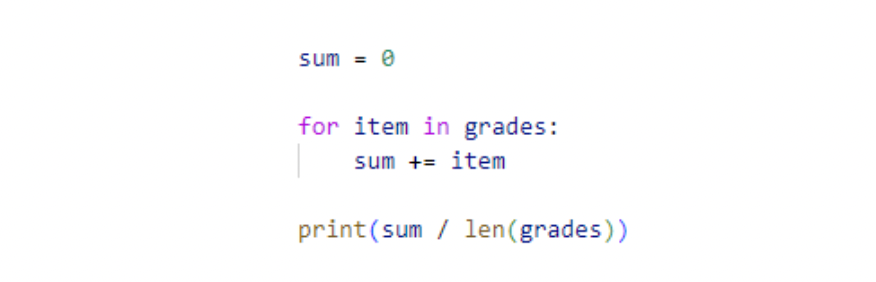
Output:

Try using Python Lists
Lists make it easier for us to work with multiple related values in our programs. Lists help us organize information and make it easy to search for information and perform calculations. You can learn more about the different ways we can use lists in our live online Python for AI course or Python Camps, designed by experts from Google and MIT!
Written by Jamila Cocchiola who has always been fascinated with technology and its impact on the world. The technologies that emerged while she was in high school showed her all the ways software could be used to connect people, so she learned how to code so she could make her own! She went on to make a career out of developing software and apps before deciding to become a teacher to help students see the importance, benefits, and fun of computer science.