Python is commonly used to develop AI applications, such as improving human to computer interactions, identifying trends, and making predictions. One way that Python is used for human to computer interactions is through chatbots. Chatbots use artificial intelligence and natural language processing to allow us to communicate with a computer more naturally. We interact with chatbots using text or voice commands when we are trying to contact customer service or when we are asking our Alexa or Google Home to answer a question or perform a task. Let's learn how to make AI in Python!
How to make your first AI in Python
Today you will learn how to make your first AI in Python using some basic techniques. Through this tutorial, you will get a basic understanding of how chatbots work. The chatbots you interact with everyday are pretty smart because they use additional algorithms and libraries. You can explore some examples of these at the bottom of this tutorial or in our live online, small-group Python for AI class, led by an expert, and designed by professionals from Google, Stanford, and MIT.
Step 1: Create a new Python program.
Let's start by accessing Replit and creating a new Python program. Click the Start Coding button on the page to sign in or create an account. You can also click the Log in or Sign up buttons in the top right corner of the website.
Once you have created an account or logged in, you can create a new Python program by clicking the Create button in the upper left corner of the page. Choose Python from the Template dropdown and give your program a name, like Python AI Chatbot.
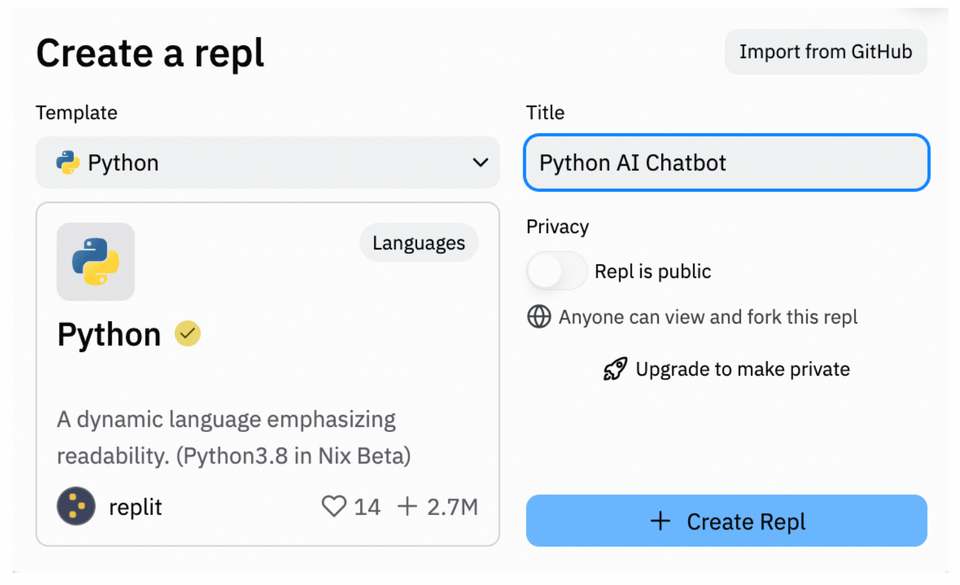
To make a simple chatbot you can follow these steps:
Step 2: Create greetings and goodbyes for your AI chatbot to use.
In Python, we can create a list that contains multiple items. Create a list called greetings and another called goodbyes. Inside a set of square brackets ( [ ] ), give your AI chatbot some greetings and goodbyes.

Step 3: Create keywords and responses that your AI chatbot will know.
Let's create a couple more lists of keywords and responses that your AI chatbot will know. Name these lists keywords and responses. Make sure the keywords and responses are in the same order. For example, if the user enters something containing the keyword "book", then the AI chatbot will respond with "I know about a lot of books." The keyword "book" and the response "I know about a lot of books" are both in the third position of both lists.

Step 4: Import the random module.
We can choose a random greeting and goodbye each time the user interacts with the AI chatbot. First, we need to import the random module to include this capability in our program. At the top of your program (first line), add:

Step 5: Greet the user.
Let's choose a random greeting from the greetings list. After the lists you created, add:

This will choose a random greeting from the greetings list and print it.
Let's also prompt the user to enter something. We can use the input function to display a prompt to the user and get their response. We also need to store their response in a variable so we can use it in our program.

The user = user.lower() converts the user's response to lowercase and stores the lowercase version of their response in the user variable.
Step 6: Keep interacting with the user until they say "bye".
We can use a while loop to keep interacting with the user as long as they have not said "bye". This while loop will repeat its block of code as long as the user response is not "bye".

Step 7: Check if the user's response contains a keyword the AI chatbot already knows.
Inside the while loop, we need to check if the user's response contains a keyword the AI chatbot already knows. We'll use a for loop to loop from the beginning to the end of the keywords list. If the keyword at the current position in the list is in the user's response, we'll print the corresponding response from the responses list.
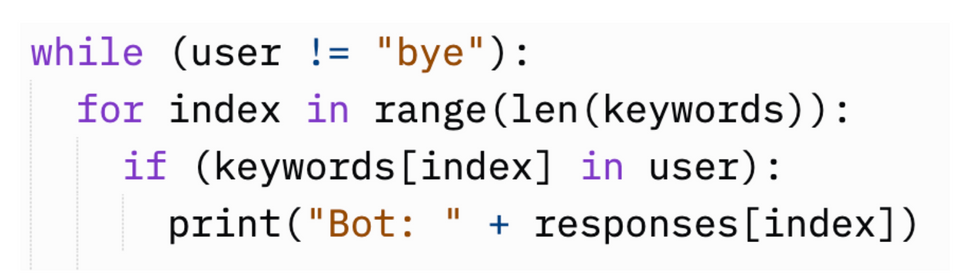
Step 8: Teach the AI chatbot a new keyword and response.
If the user's response does not contain a keyword the AI chatbot already knows, we need to teach it how to respond. Let's start by updating our while and for loops with a keyword_found variable. At the beginning of the while loop, we'll set it to false to indicate that it has not been found. In the if statement inside the for loop, we'll set the keyword_found variable to true.
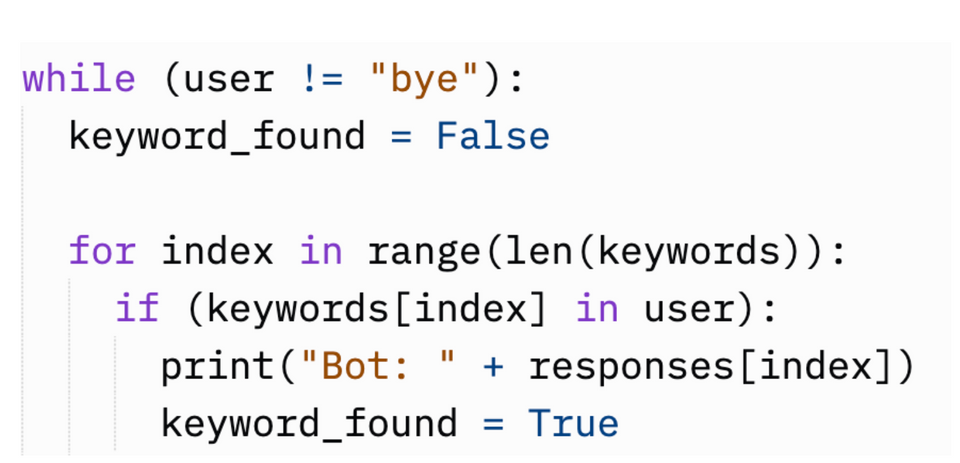
We'll add an if statement inside the while loop but outside of the for loop to check if keyword_found is false. If the user's response did not contain a keyword our AI chatbot already knew, we'll ask the user what keyword we should learn and how we should respond. We'll then add the new keyword and response to the keywords and responses lists using the append() function.
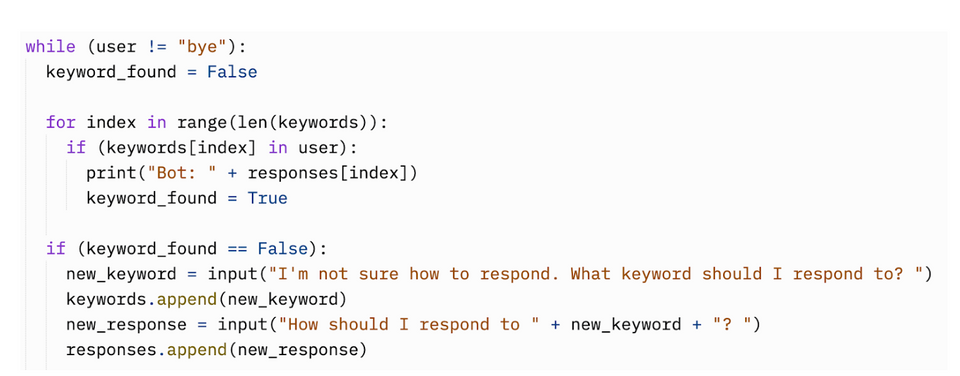
Step 9: Ask the user for another response.
At the end of the while loop, let's ask the user for another response.
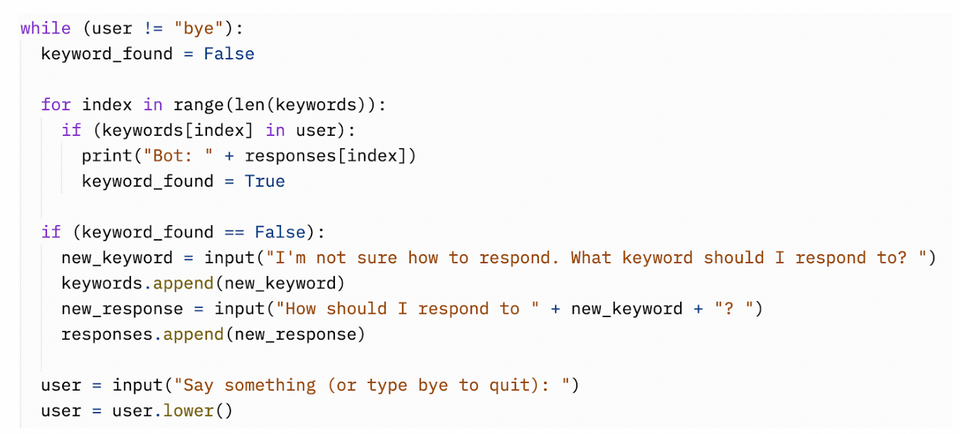
Step 10: Choose a random goodbye when the user says "bye".
If the user says "bye", the while loop will end. Outside of the while loop, let's choose a random goodbye to display to the user when they say "bye".

Congratulations! You've made your first AI in Python! You can make it smarter by adding more keywords and responses, exploring some of the libraries and project ideas listed below, or taking our Python for AI class.
Python AI source code
Take a look at the source code for this tutorial here. You can also fork this program by clicking the Fork repl button in the upper right corner to modify and add to it.
Python artificial intelligence projects for beginners
Here are some more examples of Python AI projects using additional modules to make your chatbot smarter:
- Complete Guide to build your AI Chatbot with NLP in Python: Learn about natural language processing techniques and libraries to expand your AI chatbot
- Educative's Implementing a Chatbot: Take a look at the example AI chatbot program and learn how you can train the chatbot
Make your first AI in Python
You've learned how to make your first AI in Python by making a chatbot that chooses random responses from a list and keeps track of keywords and responses it learns using lists. You can learn more about creating AI applications in Python and how to make your chatbot smarter by taking our Python camps or AI Explorers classes, designed by experts from Google, Stanford, and MIT.
Up next, explore fun Python exercises for kids.
Written by Jamila Cocchiola who has always been fascinated with technology and its impact on the world. The technologies that emerged while she was in high school showed her all the ways software could be used to connect people, so she learned how to code so she could make her own! She went on to make a career out of developing software and apps before deciding to become a teacher to help students see the importance, benefits, and fun of computer science.